Inheritance in Java: Class and Interface
Inheritance in java is a simple and powerful property in the object-oriented concept, it allows you to rewrite the attributes and methods of the parent class into the daughter class, and in this way another subclass can also inherit from the daughter class. Java uses this property in all classes.
The keyword used for inheritance in java is
extends. Multiple inheritance is forbidden, but it is allowed for interfaces.
The Object class
When programming we notice that methods are repeated everywhere such as:
toObject(),
equals(),
wait()... etc. This is because in java all classes inherit from the super class.
Object which is the root of the entire hierarchy.
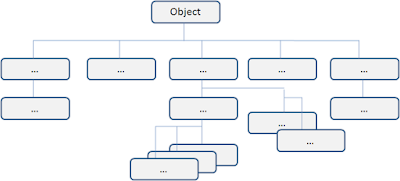 |
All classes inherit from Object |
Example
In this example, we have declared a person class with the name and address attributes. Both subclasses inherit from Person: the first class director and the second class Employee which has as an additional attribute salary.
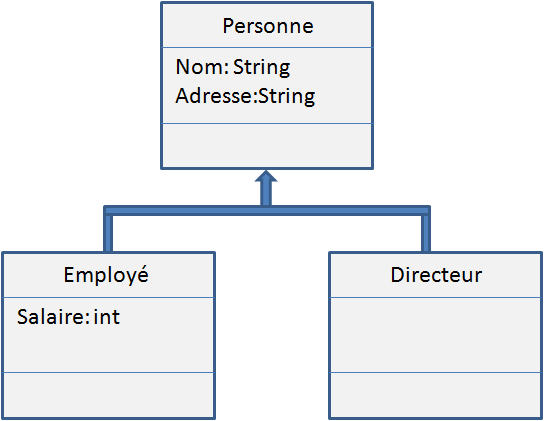
class Person
{
public String name;
public String address;
}
class Employee extends Person
{
int salary;
public Employee(String name, String address, int salary)
{
this.name=name;
this.address=address;
this.salary=salary;
}
}
class Director extends Person
{
public Director()
{
this.name= "name";
this.adresse= "address";
}
}
note:
The daughter class inherits the declared members
protected and
public and also inherits the members
private if it is in the same package as the parent class.
The super keyword in java
The Person class can be expanded to be inherited from it into subclasses, considering that in this company there are several functions: engineer, accountant, secretary... etc.
class Engineer extends Employee
{
public Engineer()
{
super("name","address",110);
}
public void concevoir(){System.out.println("I'm an engineer");}
public void developper(){};
}
class Accountant extends Employee
{
public Accountant()
{
this.name= "name";
this.adresse= "address";
this.salary = 3000;
}
public void manageAccounts(){};
public void gererLesBilans(){};
}
We see the structure in the form of a hierarchy, this tree helps us to understand the structure of our program. The difference between the two classes Engineer and Employee is the use of the super keyword in Engineer which calls the constructor of the parent class directly. By default, the
super() invokes the manufacturer without arguments and
super(p1, p2,...) invokes the constructor with arguments.
The super keyword is also used to call methods of the higher class. Here's an example:
class Ingenieur_reseaux extends Employee
{
public Ingenieur_reseaux ()
{
super("name","address",3100);
}
public void concevoir(){
super.concevoir();
System.out.println("I designed the company's network architecture");
}
}
Without super the design() method declared in the constructor Ingénieur_réseaux will be called instead of the design() method declared in engineer, so to refer to the parent method you need to add the keyword super.
Runtime:
I'm an engineer
I designed the company's network architecture
Interface inheritance in java
Multiple inheritance is allowed for interfaces.
interface print{
void print();
}
interface display{
void display();
}
interface interfaceIA extends print,display{
void imprimer_afficher();
}
class testIA implements interfaceIA{
void print(){System.out.println("Printing")};
void display(){System.out.println("Print Complete")};
public static void main(){
testIA test = new testIA();
test.print();
test.display();
}
}